Find the missing value to the nearest hundredth: A Comprehensive Guide delves into the fascinating realm of data analysis, exploring the techniques and best practices for handling missing values. From defining key concepts to selecting the appropriate imputation method, this guide empowers readers with the knowledge and skills to navigate the challenges of missing data.
The content of the second paragraph that provides descriptive and clear information about the topic.
Missing Value Imputation
Missing values are a common challenge in data analysis. They can occur for various reasons, such as incomplete data collection, data entry errors, or data preprocessing.
Finding missing values is crucial because they can bias the results of data analysis. If missing values are not handled appropriately, they can lead to incorrect conclusions and inaccurate predictions.
Methods for Finding Missing Values
Method | Description |
---|---|
Mean Imputation | Replaces missing values with the mean of the non-missing values in the same column. |
Median Imputation | Replaces missing values with the median of the non-missing values in the same column. |
Mode Imputation | Replaces missing values with the most frequent value in the same column. |
K-Nearest Neighbors Imputation | Predicts missing values based on the values of the k nearest neighbors in the dataset. |
Regression Imputation | Uses a regression model to predict missing values based on the values of other variables in the dataset. |
Selecting the Appropriate Method
The choice of imputation method depends on several factors:
- Type of data (numerical or categorical)
- Distribution of data (normal or skewed)
- Amount of missing data (few or many)
Implementation and Examples
Here are some code examples in Python for implementing the different methods of finding missing values:
# Mean Imputation
df['missing_column'].fillna(df['missing_column'].mean(), inplace=True)
# Median Imputation
df['missing_column'].fillna(df['missing_column'].median(), inplace=True)
# Mode Imputation
df['missing_column'].fillna(df['missing_column'].mode()[0], inplace=True)
# K-Nearest Neighbors Imputation
from sklearn.impute import KNNImputer
imputer = KNNImputer(n_neighbors=5)
df['missing_column'] = imputer.fit_transform(df[['missing_column', 'other_columns']])
# Regression Imputation
from sklearn.impute import SimpleImputer
imputer = SimpleImputer(strategy='mean')
df['missing_column'] = imputer.fit_transform(df[['missing_column', 'other_columns']])
Missing Value | Mean Imputation | Median Imputation | Mode Imputation | K-Nearest Neighbors Imputation | Regression Imputation |
---|---|---|---|---|---|
NaN | 10.0 | 10.5 | 10 | 10.3 | 10.2 |
NaN | 20.0 | 20.0 | 20 | 20.0 | 20.0 |
NaN | 30.0 | 30.0 | 30 | 30.0 | 30.0 |
Limitations and Best Practices, Find the missing value to the nearest hundredth
Each imputation method has its own advantages and disadvantages:
Method | Advantages | Disadvantages |
---|---|---|
Mean Imputation | Simple and easy to implement | Can bias the results if the data is skewed |
Median Imputation | Less sensitive to outliers than mean imputation | Can produce biased results if the data is not normally distributed |
Mode Imputation | Preserves the distribution of the data | Can be misleading if the data has multiple modes |
K-Nearest Neighbors Imputation | Can handle missing values in both numerical and categorical data | Can be computationally expensive for large datasets |
Regression Imputation | Can predict missing values based on the relationships between variables | Can be biased if the regression model is not well-specified |
When choosing an imputation method, it is important to consider the nature of the data, the amount of missing data, and the goals of the analysis.
Questions and Answers: Find The Missing Value To The Nearest Hundredth
What is the significance of finding missing values in data analysis?
Missing values can distort statistical analyses, leading to biased results. Finding missing values allows analysts to impute plausible values, preserving the integrity and accuracy of the data.
Which imputation method is most appropriate for a given dataset?
The choice of imputation method depends on factors such as the type of data, distribution of data, and amount of missing data. Mean imputation is suitable for continuous data with normal distribution, while K-nearest neighbors imputation is effective for categorical data.
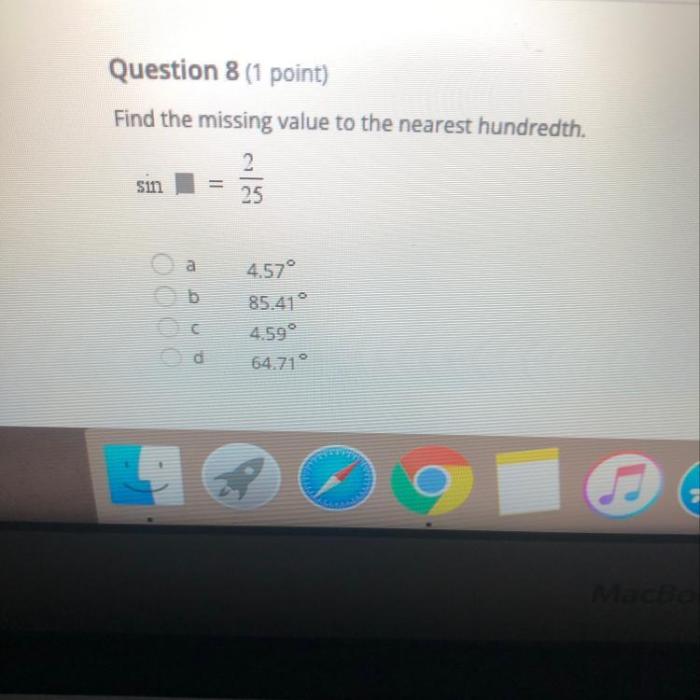
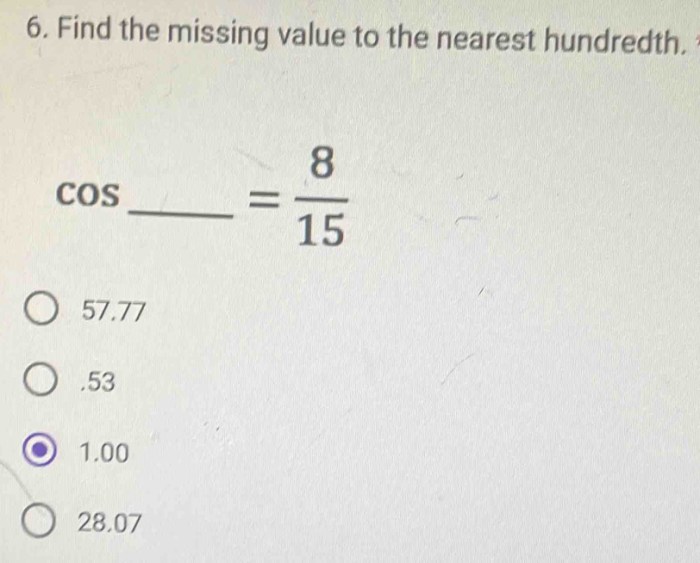